# extract apk to <target-name> folder apktool.bat d <target-name>.apk # extract classes.dex and convert dex to jar d2j-dex2jar classes.dex # convert jar class files to java jd-gui.exe classes-dex2jar.jar # analyze and hack smali files based on java files edit <critical *.smali files in <target-name> folder> # build apk (output apk located in <target-name>/dist folder) apktool.bat b <target-name> # open easy tool to sign hacked apk "APK Easy Tool.exe" # "select" <target-name>/dist/<target-name>.apk # click on "sign selected APK"
作者:yobibytes
Javascript一次性查看所有漫画图片:http://18h.mm-cg.com
在http://18h.mm-cg.com(18H,18h漫!在線H成人漫畫,18H千本H中漫與您分享)网站上一次性load所有图片:
- 在Firefox地址栏里键入”about:config”
- 把”noscript.allowURLBarJS”修改为true
- 打开漫画网页
- 在Firefox地址栏里键入”javascript:jpg_star=1; jpg_end=Large_cgurl.length+1;show_print();”
Java 7 & Java 8 changes
Java 7
Binary Literals:
-
int[] array = { 0b1111, 0b0000 };
Type Inference:
-
Map<String, String> map = new HashMap<>();
Underscores:
- double pi = 3.14159_26535;
- long lightyear = 9_460_730_472_580_800;
- long header = 0xCAFE_BABE;
String switch:
-
switch(str) { case "key1": return 1; case "key2": return 2; default: throw new NotFoundException(); }
try-with-resources:
-
try (FileReader fr = new FileReader(fileName); BufferedReader br = new BufferedReader(fr);) { br.readLine(); }
Catching multiple exception types:
-
try { method.invoke(object, new Object[] {}); } catch (IllegalArgumentException | NullPointerException ex) { logger.warn("Failed!", ex); }
Rethrowing Exceptions with type checking:
-
void method() throws Exception1, Exception2 { try { if (error1) throw new Exception1(); if (error2) throw new Exception2(); } catch (Exception e) { throw e; } }
Java 8
Lambdas (Closures):
-
Arrays.asList("a", "b", "c").forEach( e -> System.out.println( e ) );
-
Arrays.asList("a", "b", "c").forEach( (String e) -> { System.out.println( e ); System.out.println( e ); } );
-
Arrays.asList("a", "b", "c").sort( ( e1, e2) -> e1.compareTo( e2 ) );
-
Arrays.asList("a", "b", "c").sort( ( e1, e2) -> { int result = e1.compareTo( e2 ); return result; } );
Functional Interfaces:
-
@FunctionalInterface public interface Function1 { void method(); }
Default methods in interfaces:
-
public interface Defaultable { default String notRequired() { return "default implementation"; } }
Static methods in interfaces:
-
public interface DefaultableFactory { // declares and provides implementation of static methods static Defaulable create( Supplier< Defaulable > supplier) { return supplier.get(); } } public static void main(String... args) { Defaulable obj = DefaulableFactory.create( DefaultableImpl::new ); }
Method References:
-
public static class Car { // constructor reference with the syntax Class::new or Class< T > ::new public static Car create( final Supplier< Car > supplier ) { return supplier.get(); } // reference to static method with the syntax Class::static_method public static void collide( final Car car ) { ... } // reference to instance method of arbitrary object of specific type with the syntax Class::method public static void repair( ) { ... } // reference to instance method of particular class instance with the syntax instance::method public void follow( final Car another ) { ... } } public static void main(String... args) { final Car car = Car.create( Car::new ); final List< Car > cars = Arrays.asList( car); cars.forEach( Car::collide ); cars.forEach( Car::repair ); final Car police = Car.create( Car::new ); cars.forEach( police::follow ); }
Repeating annotations:
-
@Target(ElementType.TYPE) @Retention(RetentionPolicy.RUNTIME) public @interface Filters { Filter[] value(); } @Target(ElementType.TYPE) @Retention(RetentionPolicy.RUNTIME) @Repeatable(Filters.class) public @interface Filter { String value(); } @Filter("filter1") @Filter("filter2") public interface Filterable { } public static void main(String... args) { for( Filter filter: Filterable.class.getAnnotationsByType( Filter.class )) { System.out.println( filter.value() ); } }
Better Type Inference:
-
public class Value< T > { public static< T > T defaultValue() { return null; } public T getOrDefault( T value, T defaultValue ) { return ...; } } public static void main(String... args) { final Value< String > value = new Value<>(); value.getOrDefault("2", Value.defaultValue() ); }
Extended Annotations Support:
-
local variables
-
generic types
-
super-classes
-
implementing interfaces
-
exception declarations
Optional:
-
Optional<String> name = Optional.ofNullable( null );
-
name.isPresent(); // false
-
name.orElseGet( () > "none" )); // none
-
name.map( s > "Hi "+s).orElse("Hi!")); // Hi!
Stream API:
-
final Collection< Task > tasks = Arrays.asList( new Task(Tastus.OPEN, 5), ... );
-
final long totalPointsOfOpenTasks = tasks.stream() // filters out all NON-OPEN tasks .filter( task > task.getStatus() == Status.OPEN ) // converts the stream to the stream of Integers .mapToInt( Task:.getPoints ) .sum();
-
final double totalPoints = tasks.stream() // process all tasks in parallel .parallel() // converts the stream to the stream of Integers .map( task > task.getPoints() ) // calculate the final result using reduce method .reduce( 0, Integer::sum );
-
final Map<Status, List<Task>> map = tasks.stream() // group tasks by their status .collect( Collectors.groupingBy( Task::getStatus());
-
final Path path = new File(filename).toPath(); try (Stream< String> lines = Files.lines( path, StandardCharsets.UTF_8 )) { lines.onClose( () > System.out.println("Done!")).forEatch(System.out.println); }
Date/Time API:
-
Clock clock = Clock.systemUTC();
-
LocalDate date = LocalDate.now();
-
LocalTime time = LocalTime.now();
-
LocalDateTime datetime = LocalDateTime.now();
-
ZonedDateTime zonedDatetime = ZonedDateTime.now( ZoneId.of("America/Los_Angeles") );
-
Duration duration = Duration.between( from, to);
Nashorn JavaScript engine:
-
(new ScriptEngineManager()).getEngineByName("JavaScript").eval("function f() { return 1; }; f() + 1;"); // result: 2
- command line: “jjs func.js”
Base64:
-
String encoded = Base64.getEncoder().encodeToString( str.getBytes( StandardCharsets.UTF_8 ));
-
String decoded = new String( Base64.getDecoder().decode( encoded), StandardCharsets.UTF_8 )
-
// Base64.getMimeEncoder()
-
// Base64.getMimeDecoder()
-
// Base64.getUrlEncoder()
-
// Base64.getUrlDecoder()
Parallel Arrays:
-
Arrays.parallelSetAll( array, index > ThreadLocalRandom.current().nextInt( 100000) );
-
Arrays.parallelSort( array );
-
Arrays.stream( array ).limit( 10).forEach( i > System.out.print(i + " "));
JVM Metaspace:
- // replaces PermGen
- -XX:MetaSpaceSize -XX:MaxMetaspaceSize
ldapsearch的应用
在其他人公开的LDAP-属性里查找感兴趣的内容:
ldapsearch -x -LLL -W -D -b “DC=X,DC=Y,DC=com” “(sAMAccountName=)” cn l telephoneNumber mail
Create thumbnails using PDFRenderer and ImgScalr
public static boolean createThumbnail(File inputFile, File targetFile)
{
try
{
BufferedImage img = null;
if (inputFile != null && targetFile != null && inputFile.getName().toLowerCase().endsWith(".pdf"))
{
img = convertPdfToImage(inputFile);
} else
{
img = ImageIO.read(inputFile);
}
BufferedImage thumbImg = createThumbnail(img);
ImageIO.write(thumbImg, "png", targetFile);
return true;
} catch (Exception e)
{
}
return false;
}
private static BufferedImage createThumbnail(BufferedImage img)
{
BufferedImage result = null;
if (img.getWidth() < img.getHeight())
{
result = resize(img, Method.QUALITY, Mode.FIT_TO_HEIGHT, 128, OP_ANTIALIAS);
} else
{
result = resize(img, Method.QUALITY, Mode.FIT_TO_WIDTH, 128, OP_ANTIALIAS);
}
return result;
}
private static BufferedImage convertPdfToImage(File pdfFile) throws IOException
{
RandomAccessFile raf = null;
FileChannel channel = null;
try
{
raf = new RandomAccessFile(pdfFile, "r");
channel = raf.getChannel();
ByteBuffer buf = channel.map(FileChannel.MapMode.READ_ONLY, 0, channel.size());
PDFFile pdffile = new PDFFile(buf);
PDFPage page = pdffile.getPage(0);
Rectangle rect = new Rectangle(0, 0, (int) page.getBBox().getWidth(), (int) page.getBBox().getHeight());
Image image = page.getImage(rect.width, rect.height, rect, null, true, true);
BufferedImage img = new BufferedImage(rect.width, rect.height, BufferedImage.TYPE_INT_RGB);
Graphics2D bufImageGraphics = img.createGraphics();
bufImageGraphics.drawImage(image, 0, 0, null);
return img;
} finally
{
if (channel != null)
{
channel.close();
}
if (raf != null)
{
raf.close();
}
}
}
Regular Expression: detect executables
String test = "c:\\test\\test.bat";
if (test.matches("^.*\\.(exe|scr|pif|vb[es]|js|jse|ws[fh]|sh[sb]|lnk|bat|cmd|com|ht[ab])|$"))
{
System.out.println("block");
} else
{
System.out.println("pass");
}
Installing Python 2.7 on SLES 11
#!/bin/bash
# Install Python 2.7.2 alternatively
zypper install -t pattern sdk_c_c++ -y
zypper install readline-devel openssl-devel gmp-devel ncurses-devel gdbm-devel zlib-devel expat-devel libGL-devel tk tix gcc-c++ libX11-devel glibc-devel bzip2 tar tcl-devel tk-devel pkgconfig tix-devel bzip2-devel sqlite-devel autoconf db4-devel libffi-devel valgrind-devel -y
mkdir tmp
cd tmp
wget http://python.org/ftp/python/2.7.2/Python-2.7.2.tgz
tar xvfz Python-2.7.2.tgz
cd Python-2.7.2
./configure --prefix=/opt/python2.7 --enable-shared
make
make altinstall
echo "/opt/python2.7/lib" >> /etc/ld.so.conf.d/opt-python2.7.conf
ldconfig
cd ..
cd ..
rm -rf tmp
# source: https://stackoverflow.com/questions/10940296/installing-python-2-7-on-sles-11
strato DDNS VBS Client
看了几款网上的DDNS Client。有些是Shareware要付费的,有些功能不符合。最后还是写了个VBS的DDNS Client。大家可以把他添加到Windows Task Scheduler里面,让它定时通过DDNS更新external的IP。
以下是给德国Strato公司配置的(其它DDNS可见最后的Comment),在使用前按需要先修改host,pass等variables:
' === settings ===
host = "<HOST>"
pass = "<PASSWORD>"
url = "https://dyndns.strato.com/nic/update?hostname=" & host & "&system=dyndns&wildcard=OFF&backmx=NO&offline=NO"
pth = "D:\ddns-client.log"
' === ddns client ===
Dim oQuery: Set oQuery = CreateObject("MSXML2.XMLHTTP.3.0")
Dim oLogger: Set oLogger = CreateObject("Scripting.FileSystemObject")
Sub Debug (pth, str)
msg = date & " " & time & ": " & str
Set oLogFile = oLogger.OpenTextFile(pth, 8, True, 0)
oLogFile.Write msg
oLogFile.Close
End Sub
Function ResolveIP(host)
oQuery.Open "GET", "http://ip-lookup.net/domain.php?domain=" & host, False
oQuery.Send
If oQuery.Status = 200 Then
Set RegEx = New RegExp
RegEx.Global = True
RegEx.Pattern = "http://ip-lookup.net\?(\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3})"
Set matches = RegEx.Execute(oQuery.responseText)
If matches.Count > 0 Then
ResolveIP = matches(0).SubMatches(0)
End If
End If
End Function
Function GetMyIP()
oQuery.Open "GET", "https://ip.appspot.com/", False
oQuery.Send
If oQuery.Status = 200 Then
GetMyIP = Replace(Replace(oQuery.responseText, vbLf, ""), vbCr, "")
End If
End Function
Function Query(url, host, pass, ip)
If ip "" Then
url = url & "&myip=" & ip
End If
oQuery.Open "GET", url, False, host, pass
oQuery.Send
If oQuery.Status = 200 And (InStr(oQuery.responseText, "good") Or InStr(oQuery.responseText, "nochg")) Then
Query = "ok, " & oQuery.Status & ", " & oQuery.responseText
Else
Query = "failed, " & oQuery.Status
End If
End Function
' === main ===
externalIp = GetMyIP()
currentIp = ResolveIP(host)
Debug pth, host & ": current ip=" & currentIp & ", external ip=" & externalIp
If externalIp <> currentIp Then
rsp = Query(url, host, pass, ip)
Debug pth, rsp
Else
Debug pth, "ok"
End If
' === list of response codes ===
' good - Update successfully.
' nochg - Update successfully but the IP address have not changed.
' nohost - The hostname specified does not exist in this user account.
' abuse - The hostname specified is blocked for update abuse.
' notfqdn - The hostname specified is not a fully-qualified domain name.
' badauth - Authenticate failed.
' 911 - There is a problem or scheduled maintenance on provider side
' badagent - The user agent sent bad request(like HTTP method/parameters is not permitted)
' badresolv - Failed to connect to because failed to resolve provider address.
' badconn - Failed to connect to provider because connection timeout.
' === list of ddns providers ===
'DYNDNS.org
'http://members.dyndns.org/nic/update?hostname=[HOST]&myip=[IP]&system=dyndns&wildcard=NOCHG&mx=NOCHG&backmx=NOCHG
'TwoDNS.de
'http://update.twodns.de/update.php?hostname=[HOST]&myip=[IP]
'NoIP.com
'http://dynupdate.no-ip.com/nic/update?hostname=[HOST]&myip=[IP]
'able.or.kr
'http://able.or.kr/ddns/src/update.php?hostname=[HOST]&myip=[IP]&ddnsuser=[USER]&pwd=[PASS]
'3322.org
'http://www.3322.org/dyndns/update?hostname=[HOST]&system=dyndns
'selfHOST.de
'http://carol.selfhost.de/nic/update?hostname=[HOST]&myip=[IP]
'Dynamic DO!.jp
'http://free.ddo.jp/dnsupdate.php?dn=[HOST]&pw=[PASS]&ip=[IP]
'ChangeIP.com
'http://nic.ChangeIP.com/nic/update?hostname=[HOST]&myip=[IP]&system=dyndns
'DNSPod.com
'http://dnsapi.cn/Record.Modify?login_email=[USER]&login_password=[PASS]&format=xml&domain_id=[DOMAIN_ID]&record_id=[RECORD_ID]&sub_domain=[SUBDOMAIN]&record_type=A&record_line=[RECORDLINE]&value=[IP]&mx=[MX]&ttl=[TTL]
'Zoneedit.com
'http://dynamic.zoneedit.com/auth/dynamic.html?host=[HOST]&dnsto=[IP]
'Freedns.org
'http://freedns.afraid.org/dynamic/update.php?user=[FREE_DNS_SHA1]&host=[HOST]& address=[IP]
'STRATO
'http://[USER]:[PASS]@dyndns.strato.com/nic/update?hostname=[HOST]&myip=[IP]&wildcard=NOCHG&mx=NOCHG&backmx=NOCHG
设置Visual Studio界面语言
- 打开Visual Studio
- Tools\Options => Environment\International Settings
- 下载或选择英文
- 右键点击Visual Studio程序Icon
- 在target里添加”/lcid 1033″,如下
- “C:\Programme (x86)\Microsoft Visual Studio 11.0\Common7\IDE\devenv.exe” /lcid 1033
- 重新打开Visual Studio, Voilà!
取消中文Windows系统IME转换键Strg+Space
Eclipse, IntelliJ, Visual Studio等IDE里往往Strg+Space会作为Autocomplete/Autocorrection的Hotkey。而在windows系统里安装了中文IME以后这个组合键被霸占以用作为IME之间的互换。因为一个Bug这个组合键不能被修改或覆盖。
以下是把这个组合键删除的方法(适用于windows xp, vista, 7):
> regedt32
> HKEY_CURRENT_USER/Control Panel/Input Method/Hot Keys
> 选择 00000070 ("
Chinese (Traditional) IME - Ime/NonIme Toggle
")
> 把’Key Modifiers’的数值修改为’00 c0 00 00
‘
> 把’Virtual Key’的数值修改为’ff 00 00 00’
> 选择 00000010
("Chinese (Simplified) IME - Ime/NonIme Toggle
")
> 把’Key Modifiers’的数值修改为’00 c0 00 00
‘
> 把’Virtual Key’的数值修改为’ff 00 00 00’
示意图:
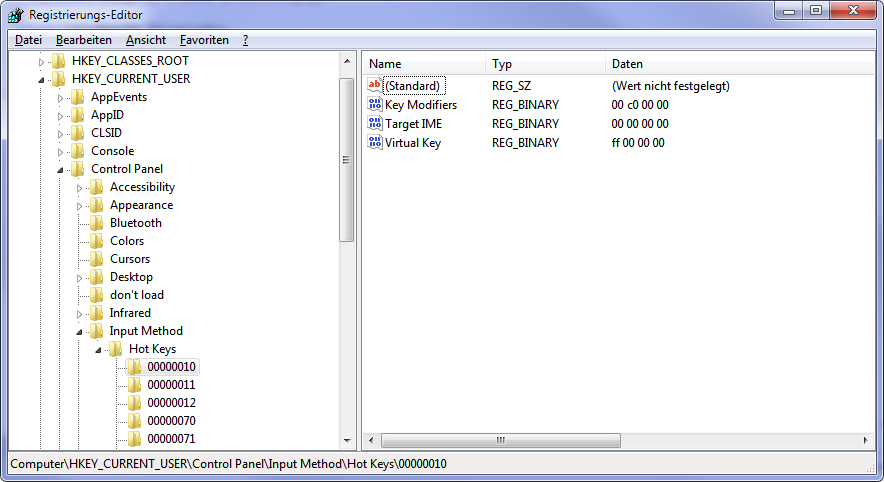